Commonly used PowerShell commands on Active Directory
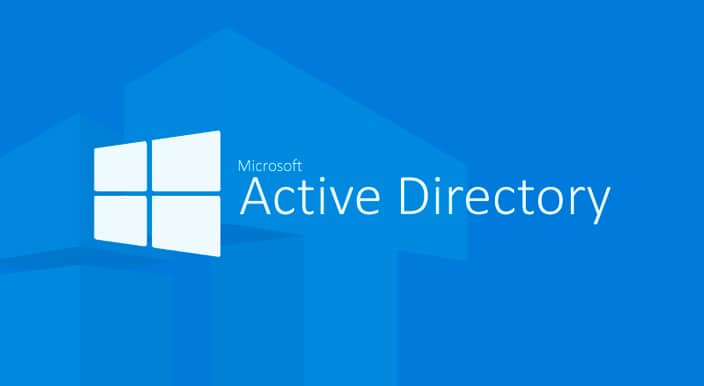
PS:
#Turn on/off protection against accidental deletion
Get-ADObject -Filter * -SearchBase "OU=Testbilgisayarlar,DC=guler,DC=com" | ForEach-Object -Process {Set-ADObject -ProtectedFromAccidentalDeletion $false -Identity $_}
#List ExtensionAttribute values in user accounts
Get-ADUser -Filter {extensionAttribute1 -notlike "*"} -SearchBase "DC=Guler,DC=com" | select name,samaccountname | Export-Csv -path "C:\extensionAttributes.csv"
Get-ADUser -Filter {extensionAttribute10 -notlike "*"} -SearchBase "DC=Guler,DC=com" | select name,samaccountname | Export-Csv -path "C:\extensionAttribute10.csv"
#AD List all Users
Get-ADUser -Filter * -Properties Name,SamAccountName,LastLogonDate | Sort | Select Name,SamAccountName,LastLogonDate,Enabled | Export-Csv "C:\allusers.csv"
#Find all users who have not logged in
Get-ADUser -Filter {(lastlogontimestamp -notlike "*")} | Select SamAccountName,Name
#Find user accounts that are enabled but have never opened an account
Get-ADUser -Filter {(lastlogontimestamp -notlike "*") -and (enabled -eq $true)} | Select SamAccountName,Name
#Fetch accounts that have not logged in for 90 days
$days = 90
$createdtime = (Get-Date).Adddays(-($days))
Get-ADUser -Filter {(lastlogontimestamp -notlike "*") -and (enabled -eq $true) -and (whencreated -lt $createdtime)} | Select SamAccountName,Name
#Find accounts that have not logged in for 30 days
$createdtime = (Get-Date).Adddays(-(30))
Get-ADUser -Filter {(lastlogontimestamp -notlike "*") -and (enabled -eq $true) -and (whencreated -lt $createdtime)} | Select Name,DistinguishedName |Export-CSV "C:\30_NeverLoggedOnUsers.csv" -NoTypeInformation -Encoding UTF8
#Enable and list those who have not logged in for 90 days
Get-ADUser -Filter {Enabled -eq $TRUE} -Properties Name,SamAccountName,LastLogonDate,Enabled | Where {($_.LastLogonDate -lt (Get-Date).AddDays(-90)) -and ($_.LastLogonDate -ne $NULL)} | Sort | Select Name,SamAccountName,LastLogonDate,Enabled | Export-Csv "C:\enable-90gun-oturum-acmayanlar.csv"
#Enable and list those who have never logged in
Get-ADUser -Filter {Enabled -eq $TRUE} -Properties Name,SamAccountName,LastLogonDate,Enabled | Where {($_.LastLogonDate -eq $NULL)} | Sort | Select Name,SamAccountName,LastLogonDate,Enabled | Export-Csv "C:\Enable_account_and_never_Login.csv"
#Find Disabled Users who have never logged in
Get-ADUser -Filter {Enabled -eq $FALSE} -Properties Name,SamAccountName,LastLogonDate,Enabled | Where {($_.LastLogonDate -eq $NULL)} | Sort | Select Name,SamAccountName,LastLogonDate,Enabled | Export-Csv "C:\newer_login_and_disable_users1.csv"
#Find Disabled users who have been disabled (logoned) in the last 180 days
Get-ADUser -Filter {Enabled -eq $FALSE} -Properties Name,SamAccountName,LastLogonDate,Enabled | Where {($_.LastLogonDate -lt (Get-Date).AddDays(-180)) -and ($_.LastLogonDate -ne $NULL)} | Sort | Select Name,SamAccountName,LastLogonDate,Enabled | Export-Csv "C:\180_days_disabled.csv"
#Find all Disabled Users
Get-ADUser -Filter {Enabled -eq $FALSE} -Properties Name,SamAccountName,LastLogonDate,Enabled | Sort | Select Name,SamAccountName,LastLogonDate,Enabled | Export-Csv "C:\all_disable_users.csv"
#AD Find All Computers
Get-ADComputer -Filter * -Properties OperatingSystem, LastLogonDate | Sort | Select Name,OperatingSystem,LastLogonDate,Enabled| Export-Csv "C:\allcomputers.csv"
#Enable Computers and find those that have not been logged in for 30 days
Get-ADComputer -Filter {Enabled -eq $TRUE} -Properties OperatingSystem, LastLogonDate | Where { $_.LastLogonDate -lt (Get-Date).AddDays(-30) } | Sort | Select Name,OperatingSystem,LastLogonDate,Enabled| Export-Csv "C:\inactivecomputers1.csv"
#Enable Computers and find those that have never been logged in
Get-ADComputer -Filter {Enabled -eq $TRUE} -Properties OperatingSystem, LastLogonDate | Where { ($_.LastLogonDate -eq $NULL) }| Sort | Select Name,OperatingSystem,LastLogonDate,Enabled| Export-Csv "C:\enable_and_NeverLoginComp.csv"
#All Disable Computers
Get-ADComputer -Filter {Enabled -eq $FALSE} -Properties OperatingSystem, LastLogonDate | Sort | Select Name,OperatingSystem,LastLogonDate,Enabled| Export-Csv "C:\disabled_computers.csv"
#All about Domain Controllers - Whoami
$domain = [System.Directoryservices.Activedirectory.Domain]::GetCurrentDomain()
$domain | ForEach-Object {$_.DomainControllers} |
ForEach-Object {
$hostEntry= [System.Net.Dns]::GetHostByName($_.Name)
New-Object -TypeName PSObject -Property @{
Name = $_.Name
IPAddress = $hostEntry.AddressList[0].IPAddressToString
}
} | Export-CSV "C:\domaincontrollers.csv" -NoTypeInformation -Encoding UTF8
#Find DHCP Servers
netsh dhcp show server | Export-Csv "C:\alldhcpserverlist.csv"
#Move user to a different OU
Get-ADUser $name| Move-ADObject -TargetPath 'OU=users,DC=Guler,Dc=com'
Get-ADUser “faruk” | Move-ADObject -TargetPath “OU=Uyeler,DC=Guler,DC=com”
#Get total number of users
(Get-ADUser -filter *).count
#Get the number of computers in Active Directory
(Get-ADComputer -Filter *).Count
#Find the number of users in a particular OU
(Get-ADUser -filter * -searchbase "OU=personel, OU=uyeler, DC=Guler, DC=com").count
#Get the number of enabled users
(Get-AdUser -filter * |Where {$_.enabled -eq "True"}).count
#Get the number of disabled users
(Get-ADUser -filter * |Where {$_.enabled -ne "False"}).count
#Get the number of users in the group
(Get-ADGroupMember -Identity "Group Name").count
#List Shared Mailboxes on Exchange Server
Get-Recipient -Resultsize unlimited | where {$_.RecipientTypeDetails -eq "SharedMailbox"} | ft Name,Manager >C:\shared_mailboxes.csv
#List Contacts in Exchange Server
Get-Recipient -Resultsize unlimited | where {$_.RecipientTypeDetails -eq "Contacts"} | ft Name,Manager >C:\all_contacts.csv
#Deleting a specific User
#Remove-ADUser -identity "faruk" -Confirm:$False
#Find Computers Inactive for 100 days
$DaysInactive = 100
$time = (Get-Date).Adddays(-($DaysInactive))
Get-ADComputer -Filter {LastLogonTimeStamp -lt $time} -ResultPageSize 99999 -resultSetSize $null -Properties Name, OperatingSystem, SamAccountName, DistinguishedName | select Name
Saygılarımla – Best Regards
This post is licensed under CC BY 4.0 by the author.